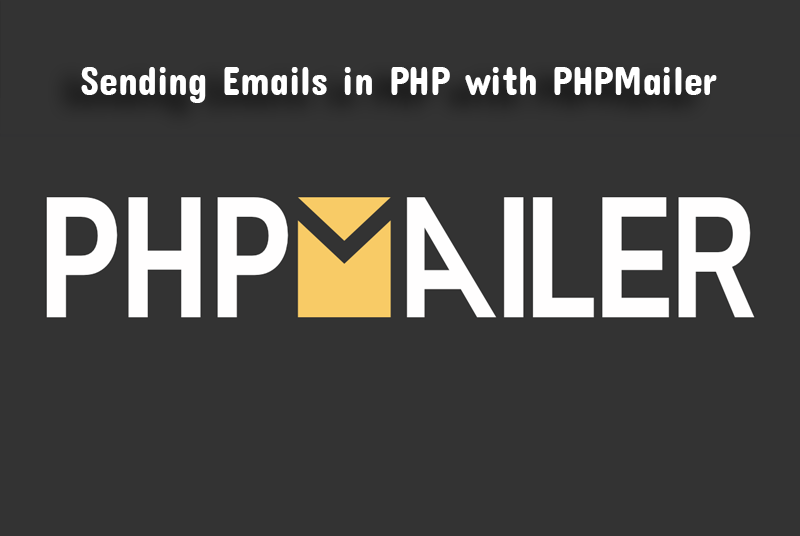
Sending Emails in PHP with PHPMailer
This entry was posted on Friday January 17, 2020PHPMailer is one of the most notable open source PHP libraries to send messages with. It was first released course in 2001 and starting now and into the foreseeable future it has become a PHP originator’s favored technique for sending messages consequently, adjacent to two or three other fan top decisions like Swiftmailer.
In this article we’ll talk about why you ought to use PHPMailer instead of PHP’s mail() limit and we’ll reveal to some code tests on the most ideal approach to use this library.
Is it an alternative as opposed to PHP’s mail() work?
A great part of the time, it’s an alternative as opposed to PHP’s mail() work, anyway there are various circumstances where the mail() work is basically not versatile enough to achieve what you need.
As an issue of first significance, PHPMailer gives a thing arranged interface, however mail() isn’t address masterminded. PHP designs generally lean toward not to make $headers strings while sending messages using the mail() work since they require a huge amount of escaping – PHPMailer makes this a breeze. Architects moreover need to make chaotic code (escaping characters, encoding and organizing) to send associations and HTML based messages while using the mail() work however PHPMailer makes this easy.
Also, the mail() work requires a local mail server to pass on messages. PHPMailer can use a non-neighborhood mail server (SMTP) if you have check.
Further central focuses include:
It can print various sorts of mix-ups messages more than 40 lingos when it fails to send an email
Facilitated SMTP show sponsorship and affirmation over SSL and TLS
Can send elective plaintext version of email for non-HTML email clients
Dynamic architect arrange which keeps awake with the most recent
PHPMailer is in like manner used by popular PHP content organization systems like WordPress, Drupal, Joomla, etc.
Introducing PHPMailer
You can introduce PHPMailer utilizing Composer:
arranger require phpmailer/phpmailer
Sending Email from Local Web Server utilizing PHPMailer
Here is the easiest case of sending an email from a nearby web server utilizing PHPMailer
<?php
require_once “vendor/autoload.php”;
//PHPMailer Object
$mail = new PHPMailer;
//From email address and name
$mail->From = “info@cilected.com”;
$mail->FromName = “Full Name”;
//To address and name
$mail->addAddress(“recepient1@cilected.com”, “Recepient Name”);
$mail->addAddress(“recepient1@cilected.com”); //Recipient name is optional
//Address to which recipient will reply
$mail->addReplyTo(“reply@cilected.com”, “Reply”);
//CC and BCC
$mail->addCC(“cc@cilected.com”);
$mail->addBCC(“bcc@cilected.com”);
//Send HTML or Plain Text email
$mail->isHTML(true);
$mail->Subject = “Subject Text”;
$mail->Body = “<i>Mail body in HTML</i>”;
$mail->AltBody = “This is the plain text version of the email content”;
if(!$mail->send())
{
echo “Mailer Error: ” . $mail->ErrorInfo;
}
else
{
echo “Message has been sent successfully”;
} ?>
The code and comments should be sufficiently clear to explain everything that’s going on.
Sending an E-Mail with Attachments
Let’s see an example on how to send an email with attachments using PHPMailer.
<?php
require_once “vendor/autoload.php”;
$mail = new PHPMailer;
$mail->From = “from@yourdomain.com”;
$mail->FromName = “Full Name”;
$mail->addAddress(“recipient1@cilected.com”, “Recipient Name”);
//Provide file path and name of the attachments
$mail->addAttachment(“file.txt”, “File.txt”);
$mail->addAttachment(“images/profile.png”); //Filename is optional
$mail->isHTML(true);
$mail->Subject = “Subject Text”;
$mail->Body = “<i>Mail body in HTML</i>”;
$mail->AltBody = “This is the plain text version of the email content”;
if(!$mail->send())
{
echo “Mailer Error: ” . $mail->ErrorInfo;
}
else
{
echo “Message has been sent successfully”;
} ?>
Here we are appending two documents i.e., file.txt which lives in a similar registry as the content and pictures/profile.png which lives in pictures catalog of the content index.
Report Advertisement
To add connections to the email we simply need to call the capacity addAttachment of the PHPMailer object by passing the record way as contention. For joining numerous documents we have to consider it on different occasions.
Utilizing SMTP
You can utilize the mail server of an another host to send email, yet for this you first need to have verification. For instance: to send an email from Gmail’s mail server you have to have a Gmail account.
SMTP is a convention utilized via mail customers to send an email send solicitation to a mail server. When the mail server checks the email it sends it to the goal mail server.
Here is a case of sending an email from Gmail’s mail server from your area. You needn’t bother with a neighborhood mail server to run the code. We will utilize the SMTP convention:
<?php
require_once “vendor/autoload.php”;
$mail = new PHPMailer;
//Enable SMTP debugging.
$mail->SMTPDebug = 3;
//Set PHPMailer to use SMTP.
$mail->isSMTP();
//Set SMTP host name
$mail->Host = “smtp.gmail.com”;
//Set this to true if SMTP host requires authentication to send email
$mail->SMTPAuth = true;
//Provide username and password
$mail->Username = “name@gmail.com”;
$mail->Password = “super_secret_password”;
//If SMTP requires TLS encryption then set it
$mail->SMTPSecure = “tls”;
//Set TCP port to connect to
$mail->Port = 587;
$mail->From = “name@gmail.com”;
$mail->FromName = “Full Name”;
$mail->addAddress(“name@cilected.com”, “Recepient Name”);
$mail->isHTML(true);
$mail->Subject = “Subject Text”;
$mail->Body = “<i>Mail body in HTML</i>”;
$mail->AltBody = “This is the plain text version of the email content”;
if(!$mail->send())
{
echo “Mailer Error: ” . $mail->ErrorInfo;
}
else {
echo “Message has been sent successfully”;
} ?>
Gmail requires TLS encryption over SMTP so we set it in like manner. Before you send by means of SMTP, you have to discover the host name, port number, encryption type whenever required and if confirmation is required you additionally need the username and secret phrase. Note that having a two-factor verification empowered on Gmail won’t let you utilize their SMTP with username/secret phrase – rather, extra design will be required.
One major favorable position in utilizing remote SMTP over nearby mail is that on the off chance that you utilize PHP’s mail() capacity to send email with the from address space set to something besides the neighborhood area (name of the server), at that point the beneficiary’s email server’s assault channels will stamp it as spam. For instance, in the event that you send an email from a server with genuine host name cilected.com with the from address name@gmail.com to name@yahoo.com, at that point Yahoo’s servers will stamp it as spam or show a message to the client not to confide in the email on the grounds that the mail’s starting point is cilected.com but it presents itself as though originating from gmail.com. In spite of the fact that you possess name@gmail.com, there is no chance to get for Yahoo to locate that out.
Recovering E-Mails utilizing POP3
PHPMailer additionally permits POP-before-SMTP confirmation to send messages. As such, you can verify utilizing POP and send email utilizing SMTP. Unfortunately, PHPMailer doesn’t bolster recovering messages from mail servers utilizing the POP3 convention. It’s constrained to just sending messages.
Showing Localized Error Messages
$mail->ErrorInfo can return blunder messages in 43 unique dialects.
To show mistake messages in an alternate language, duplicate the language catalog from PHPMailer’s source code to the venture registry.
To return mistake messages in, for instance, Russian, set the PHPMailer article to the Russian language utilizing the beneath strategy call:
$mail->setLanguage(“ru”);
You can also add your own language files to the language directory.
Conclusion
In the event that you are a PHP designer, there is minimal possibility of abstaining from sending messages automatically. While you may select outsider administrations like Mandrill or Sendgrid, once in a while that simply isn’t a choice, and rolling your very own email sending library even less so. That is the place PHPMailer and its other options (Zend Mail, Swiftmailer, and so forth..) come in.
You can find out about this current library’s APIs in the official documentation. Do you use PHPMailer? Or then again do you rather depend on completely remote API based arrangements? Tell us in the remarks!
Is it true that you are getting stalled with PHP library conditions? Watch our screen cast and find out about how Composer can assist you with dealing with this for you.