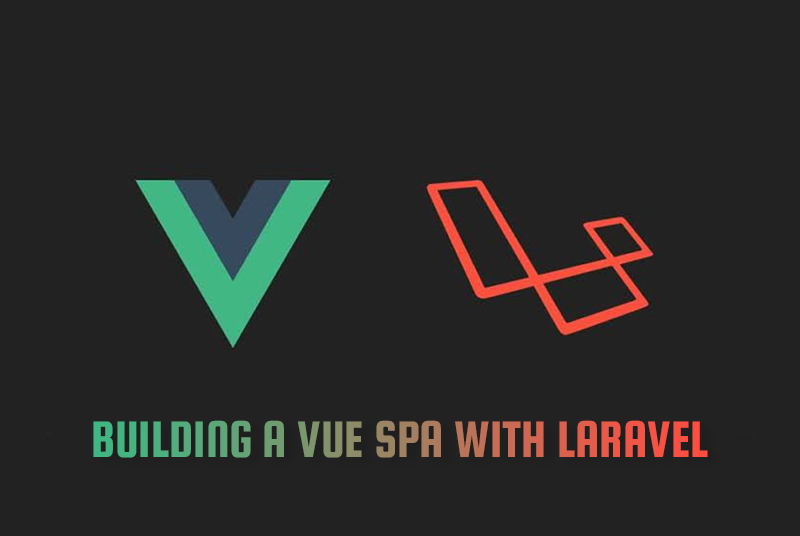
Building a Vue SPA with Laravel
This entry was posted on Friday February 7, 2020Building a Vue single page application (SPA) with Laravel is an excellent blend for building clean API-driven applications. Right now, tell you the best way to find a workable pace with Vue switch and a Laravel backend for building a SPA. We will concentrate on the wiring up every one of the pieces required, and afterward in a subsequent instructional exercise, we will additionally exhibit utilizing Laravel as the API layer.
The essential progression of how a Vue SPA functions with Laravel as a backend is as per the following:
The main solicitation hits the server-side Laravel switch
Laravel renders the SPA design
Resulting demands influence the history.pushState API for URL route without a page reload
Vue switch can be arranged to utilize history mode or the default hash-mode, which utilizes the URL hash to recreate a full URL so the page won’t reload when the URL changes.
We will utilize history mode, which implies we have to arrange a Laravel course that will coordinate every single imaginable Url relying upon which course the client enters the Vue SPA. For instance, if the client invigorates a/hi course, we’ll have to coordinate that course and return the Vue SPA application layout. The Vue Router will at that point decide the course and render the suitable segment.
Installation
To begin, we will make another Laravel extend and afterward introduce the Vue switch NPM bundle:
laravel new vue-router
cd vue-router
# Link the project if you use Valet
valet link
# Install NPM dependencies and add vue-router
yarn install
yarn add vue-router # or npm install vue-router
We have a Laravel establishment and the vue-switch NPM bundle all set. Next, we’ll design the switch and characterize a few courses and parts.
Arranging Vue Router
The manner in which that Vue Router works is that it maps a course to a Vue part and afterward renders it inside this tag in the application:
<router-view></router-view>
The switch see is inside the setting of the Vue application part that encompasses the whole application. We will return to the App part immediately.
To start with, we are going to refresh the fundamental JavaScript document assets/resources/js/app.js and design Vue switch. Supplant the substance of the app.js document with the accompanying:
import Vue from ‘vue’
import VueRouter from ‘vue-router’
Vue.use(VueRouter)
import App from ‘./views/App’
import Hello from ‘./views/Hello’
import Home from ‘./views/Home’
const router = new VueRouter({
mode: ‘history’,
routes: [
{
path: ‘/’,
name: ‘home’,
component: Home
},
{
path: ‘/hello’,
name: ‘hello’,
component: Hello,
},
],
});
const app = new Vue({
el: ‘#app’,
components: { App },
router,
});
We have a couple of records that we have to make, on the whole, we should cover the substance of app.js:
We import and introduce the VueRouter module with Vue.use()
We import three Vue segments:
an App segment that is the peripheral application segment,
A Hello component that maps to the /hello route
A Home component that maps to the /home route
We develop another VueRouter case that takes a setup object
We make Vue mindful of the App segment by passing it to the parts property in the Vue constructor
We infuse the switch consistent into the new Vue application to gain admittance to this.$router and this.$route
The VueRouter constructor takes a variety of courses, where we characterize the way, the name (simply like Laravel’s named course), and the part that maps to the way.
I like to move my course definitions into a different courses module that I import, yet for effortlessness we’ll characterize the courses inside the principle application record.
All together for Laravel blend to run effectively, we have to characterize the three parts:
mkdir resources/assets/js/views
touch resources/assets/js/views/App.vue
touch resources/assets/js/views/Home.vue
touch resources/assets/js/views/Hello.vue
First, the App.vue file is the outermost container element for our application. Inside this component, we’ll define an application heading and some navigation using Vue Router’s <router-link/> tag:
<template>
<div>
<h1>Vue Router Demo App</h1>
<p>
<router-link :to=”{ name: ‘home’ }”>Home</router-link> |
<router-link :to=”{ name: ‘hello’ }”>Hello World</router-link>
</p>
<div class=”container”>
<router-view></router-view>
</div>
</div>
</template>
<script>
export default {}
</script>
The most important tag in our App component is the <router-view></router-view> tag, which is where our router will render the given component that matches the route (i.e. Home or Hello).
The next component we need to define is located at resources/assets/js/views/Home.vue:
<template>
<p>This is the homepage</p>
</template>
Lastly, we define the Hello component located at resources/assets/js/views/Hello.vue:
<template>
<p>Hello World!</p>
</template>
I like isolating my reusable segments from my view-explicit parts by sorting out my perspectives into the assets/resources/js/sees envelope and my genuinely reusable segments in assets/resources/js/segments. This is my show, and I discover it functions admirably so I can without much of a stretch separate which parts are planned to be reusable and which segments are see explicit.
We have all that we have to run our Vue application most definitely! Next, we have to characterize the backend course and the server-side format.
The Server-Side
We influence an application system like Laravel with a Vue SPA so we can fabricate a server-side API to work with our application. We can likewise utilize Blade to render our application and uncover condition arrangement through a worldwide JavaScript object, which is helpful as I would like to think.
Right now, won’t work out an API, however we will in a development. This post is tied in with wiring up the Vue switch.
The primary thing we’ll handle on the server-side is characterizing the course. Open the courses/web.php document and supplant the welcome course with the accompanying:
<?php
/*
|————————————————————————–
| Web Routes
|————————————————————————–
|
| Here is where you can register web routes for your application. These
| routes are loaded by the RouteServiceProvider within a group which
| contains the “web” middleware group. Now create something great!
|
*/
Route::get(‘/{any}’, ‘SpaController@index’)->where(‘any’, ‘.*’);
We define a catch-all route to the SpaController which means that any web route will map to our SPA. If we didn’t do this, and the user made a request to /hello, Laravel would respond with a 404.
Next, we need to create the SpaController and define the view:
php artisan make:controller SpaController
Open the SpaController and enter the following:
<?php
namespace App\Http\Controllers;
use Illuminate\Http\Request;
class SpaController extends Controller
{
public function index()
{
return view(‘spa’);
}
}
Lastly, enter the following in resources/views/spa.blade.php:
<!DOCTYPE html>
<html lang=”en”>
<head>
<meta charset=”UTF-8″>
<meta name=”viewport” content=”width=device-width, initial-scale=1.0″>
<meta http-equiv=”X-UA-Compatible” content=”ie=edge”>
<title>Vue SPA Demo</title>
</head>
<body>
<div id=”app”>
<app></app>
</div>
<script src=”{{ mix(‘js/app.js’) }}”></script>
</body>
</html>
We’ve defined the required #app element which contains the App component that Vue will render, along with rendering the appropriate component based on the URL.
Running the Application
The foundation is in place for building an SPA with Vue and Vue Router. We need to build or JavaScript to test it out:
yarn watch # or npm run watch
If you load up the application in your browser you should see something like the following:
Going Forward
We have the skeleton for a Vue SPA that we can start building using Laravel as the API layer. This app still has much to be desired that we will cover in a follow-up tutorial:
Define a catch-all 404 route on the frontend
Using route parameters
Child routes
Making an API request from a component to Laravel
Probably much more that I’m not going to list here…