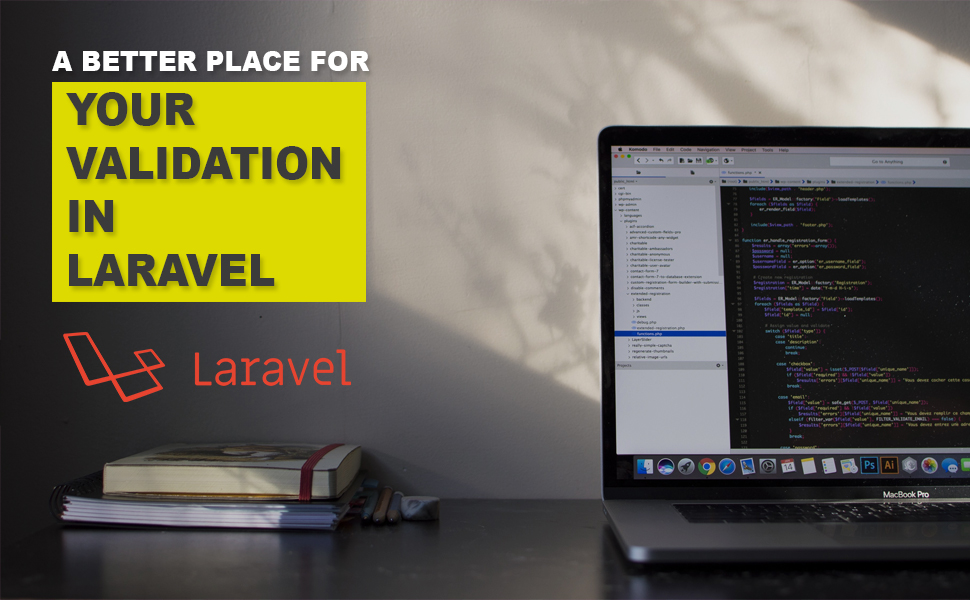
A better place for your validation in Laravel
This entry was posted on Friday December 20, 2019Approval in Laravel is truly simple. It essentially gives both of you choices where to approve an approaching solicitation:
- inside a controller activity
- via a devoted Form Request
In-Action validation
<?php namespace App\Http\Controllers; | |
use App\Http\Controllers\Controller; | |
class PostController extends Controller | |
{ | |
public function store(Request $request) | |
{ | |
$validatedData = $request->validate([ | |
‘title’ => ‘required|unique:posts|max:255’, | |
‘body’ => ‘required’, | |
]); | |
// The blog post is valid… | |
} | |
} |
This is a quite basic yet incredible methodology. You simply request that the solicitation approve itself dependent on the passed standards. In the event that the approval comes up short, the technique makes a legitimate blunder message and send it back to the view. In the event that the approval passes, it just proceeds onward with the remainder of the code inside the activity.
This can get exceptionally expressive in the event that you approve a long structure with in excess of 10 fields; yet there is something we can do about.
Form Request Validation
Validate via Form Request.
<?php namespace App\Http\Requests; | |
use Illuminate\Foundation\Http\FormRequest; | |
class PostStoreRequest extends FormRequest | |
{ | |
public function authorize() | |
{ | |
return true; | |
} | |
public function rules() | |
{ | |
return [ | |
‘title’ => ‘required|unique:posts|max:255’, | |
‘body’ => ‘required’, | |
]; | |
} | |
} |
PostsController with Form Request.
<?php namespace App\Http\Controllers; | |
use App\Http\Controllers\Controller; | |
use App\Http\Requests\SingingCircleRequest; | |
class PostsController extends Controller | |
{ | |
public function store(PostStoreRequest $request) | |
{ | |
// You can just pull the data out of PostStoreRequest | |
} | |
} |
Here we need to create a Form Request object with a meaningful name.
$ php artisan make:request PostStoreRequest
Then, we define the rules in rules() and pass the Form Request object to the action. We are now able to get the validated data out of PostStoreRequest.
I am utilizing In-Action approval for quick advancement. I like to see the principles near the code. In this stage I have to change them rapidly without fiddling a lot with a few documents.
In later state, I for the most part move them to a FormRequest class. The advantages are:
- cleaner and shorter activity
- if the approval comes up short, the activity will never hit
- the Form Request object has a devoted spot to approve the activity
Be that as it may, where light is, is additionally some dimness. The drawbacks are:
- a Form Request object for any activity who handles client input
- your approval rules are spread and are covered up in every one of these records
While the main point is much the same as it is, the last point can be tended to.
Move the rules to the model
Since we attempt to approve and store a blog entry, we likewise have a BlogPost model. Fine! Presently, simply move the standards from any place they are to the model and store them in a static variable:
<?php namespace App\Models; | |
use Illuminate\Database\Eloquent\Model; | |
class BlogPosts extends Model | |
{ | |
public static $createRules = [ | |
‘title’ => ‘required|unique:posts|max:255’, | |
‘body’ => ‘required’, | |
]; | |
public static $updateRules = [ | |
‘title’ => ‘required|unique:posts|max:255’, | |
‘body’ => ‘required’, | |
]; | |
} |
<?php namespace App\Http\Controllers; | |
use App\Http\Controllers\Controller; | |
use App\Models\BlogsPost; | |
class PostsController extends Controller | |
{ | |
public function store(Request $request) | |
{ | |
$validatedData = $request->validate(BlogsPost::$createRules); | |
// The blog post is valid… | |
} | |
} |
<?php namespace App\Http\Requests; | |
use App\Models\BlogsPost; | |
use Illuminate\Foundation\Http\FormRequest; | |
class PostStoreRequest extends FormRequest | |
{ | |
public function rules() | |
{ | |
return BlogPost::$createRules | |
} | |
} |
You can even go one step further and combine two or more rules together.
<?php namespace App\Http\Requests; | |
use App\Models\BlogsPost; | |
use Illuminate\Foundation\Http\FormRequest; | |
class PostStoreRequest extends FormRequest | |
{ | |
public function rules() | |
{ | |
return array_merge(BlogPost::$createRules, Author::$createRules); | |
} | |
} |
That is all, people. If it’s not too much trouble let me know, your opinion of this methodology via the mail.